LeetCode: 2236. Root Equals Sum of Children
The Problem
You are given the root
of a binary tree that consists of exactly 3
nodes: the root, its left child, and its right child.
Return true
if the value of the root is equal to the sum of the values of its two children, or false
otherwise.
Example
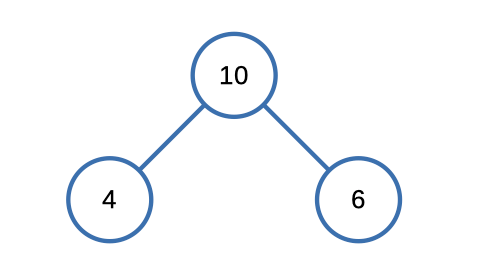
Input: root = [10,4,6]
Output: true
Explanation: The values of the root, its left child, and its right child are 10, 4, and 6, respectively.
10 is equal to 4 + 6, so we return true.
Constraints:
- The tree consists only of the root, its left child, and its right child.
-100 <= Node.val <= 100
Solution
We opted for straightforward approach to tackle this problem, and upon evaluating our solution on the LeetCode platform, we achieved the following outcome:
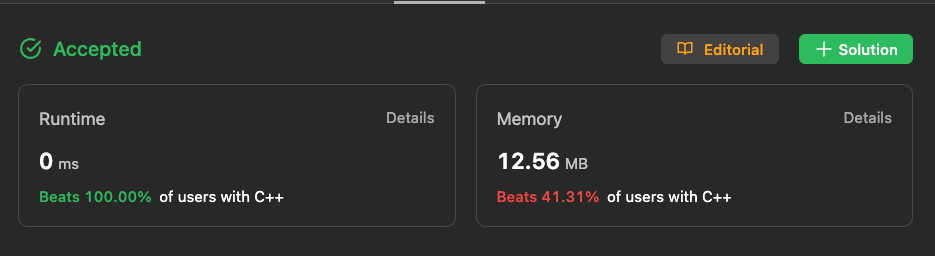
Here's the code that led us to this result.
bool checkTree(TreeNode* root) {
return root->val == (root->left->val + root->right->val);
}
Let's break down the code:
The code checks whether the value of the current node (root->val
) is equal to the sum of the values of its left and right child nodes (root->left->val + root->right->val
).
If this condition is true, the function returns true
, indicating that the condition is met for this node. Otherwise, it returns false
, indicating that the condition is not satisfied.