LeetCode: 13. Roman to Integer
The Problem
Roman numerals are represented by seven different symbols: I
, V
, X
, L
, C
, D
and M
.
Symbol ValueI 1 V 5 X 10 L 50 C 100 D 500 M 1000
For example, 2
is written as II
in Roman numeral, just two ones added together. 12
is written as XII
, which is simply X + II
. The number 27
is written as XXVII
, which is XX + V + II
.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not IIII
. Instead, the number four is written as IV
. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as IX
. There are six instances where subtraction is used:
I
can be placed beforeV
(5) andX
(10) to make 4 and 9.X
can be placed beforeL
(50) andC
(100) to make 40 and 90.C
can be placed beforeD
(500) andM
(1000) to make 400 and 900.
Given a roman numeral, convert it to an integer.
Examples
Example 1:
Input: s = "III" Output: 3 Explanation: III = 3.
Example 2:
Input: s = "LVIII" Output: 58 Explanation: L = 50, V= 5, III = 3.
Example 3:
Input: s = "MCMXCIV" Output: 1994 Explanation: M = 1000, CM = 900, XC = 90 and IV = 4.
Constraints:
1 <= s.length <= 15
s
contains only the characters('I', 'V', 'X', 'L', 'C', 'D', 'M')
.- It is guaranteed that
s
is a valid roman numeral in the range[1, 3999]
.
The Solution
We opted for a simple approach using a for loop, this was the result:
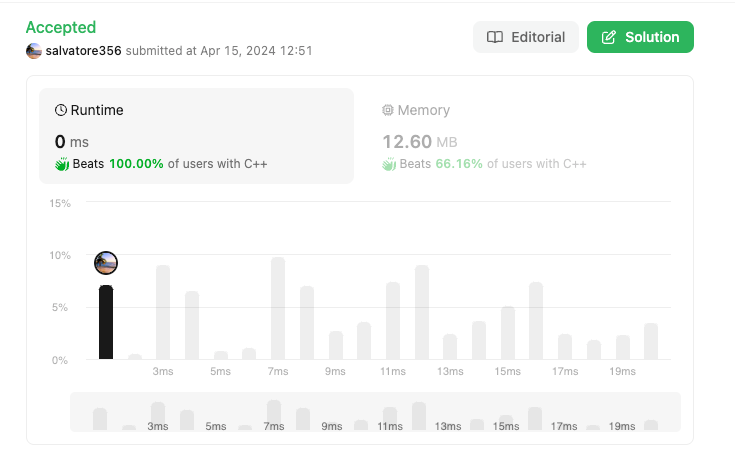
unordered_map<char, int> values = {
{'I', 1}, {'V', 5}, {'X', 10}, {'L', 50}, {'C', 100}, {'D', 500}, {'M', 1000}
};
int romanToInt(string s) {
int currentValue, lastValue = values[s.back()];
int ans = lastValue;
for(int i = s.size() - 2; i >= 0; --i) {
currentValue = values[s[i]];
if (currentValue >= lastValue) ans += currentValue;
else ans -= currentValue;
lastValue = currentValue;
}
return ans;
}
Let's break down the code step by step:
unordered_map<char, int> values = {...}:
- This line declares an unordered map named
values
which maps characters (Roman numerals) to their corresponding integer values. - Each key-value pair represents a Roman numeral character and its corresponding integer value.
- For example, 'I' maps to 1, 'V' maps to 5, 'X' maps to 10, and so on.
int romanToInt(string s):
- This line defines a function named
romanToInt
that takes a strings
as input and returns an integer.
int currentValue, lastValue = values[s.back()];:
- This line declares two integer variables
currentValue
andlastValue
. lastValue
is initialized with the integer value corresponding to the last character of the input strings
.- For instance, if
s
is "IX", thenlastValue
will be initialized with the integer value corresponding to 'X'.
int ans = lastValue;:
- This line initializes the variable
ans
with the value oflastValue
. ans
will hold the final result of converting the Roman numeral string to an integer.
for(int i = s.size() - 2; i >= 0; --i) { ... }:
- This is a
for
loop that iterates over the characters of the input strings
in reverse order, starting from the second-to-last character. - The loop continues until it reaches the first character of the string.
currentValue = values[s[i]];:
- Inside the loop,
currentValue
is assigned the integer value corresponding to the character at indexi
in the strings
. - For example, if
s[i]
is 'I', thencurrentValue
will be assigned 1.
if (currentValue >= lastValue) ans += currentValue; else ans -= currentValue;:
- This
if
statement checks whether the current integer value (currentValue
) is greater than or equal to the previous integer value (lastValue
). - If
currentValue
is greater than or equal tolastValue
, it addscurrentValue
toans
. - Otherwise, it subtracts
currentValue
fromans
. - This logic is based on the rule of Roman numerals where smaller numerals to the right of larger ones are added, and smaller numerals to the left of larger ones are subtracted.
lastValue = currentValue;:
- This line updates
lastValue
with the value ofcurrentValue
for the next iteration of the loop.
return ans;:
- Finally, the function returns the calculated integer value
ans
, which represents the conversion of the Roman numeral strings
to an integer.