LeetCode: 116. Populating Next Right Pointers in Each Node
The Problem
You are given a perfect binary tree where all leaves are on the same level, and every parent has two children. The binary tree has the following definition:
struct Node {
int val;
Node *left;
Node *right;
Node *next;
}
Populate each next pointer to point to its next right node. If there is no next right node, the next pointer should be set to NULL
.
Initially, all next pointers are set to NULL
.
Example
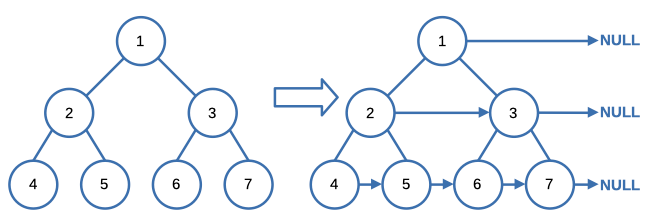
Input: root = [1,2,3,4,5,6,7]
Output: [1,#,2,3,#,4,5,6,7,#]
Explanation: Given the above perfect binary tree (Figure A), your function should populate each next pointer to point to its next right node, just like in Figure B. The serialized output is in level order as connected by the next pointers, with '#' signifying the end of each level.
Constraints:
- The number of nodes in the tree is in the range
[0, 212 - 1]
. -1000 <= Node.val <= 1000
Solution
We opted for a recursive approach to tackle this problem, and upon evaluating our solution on the LeetCode platform, we achieved the following outcome:
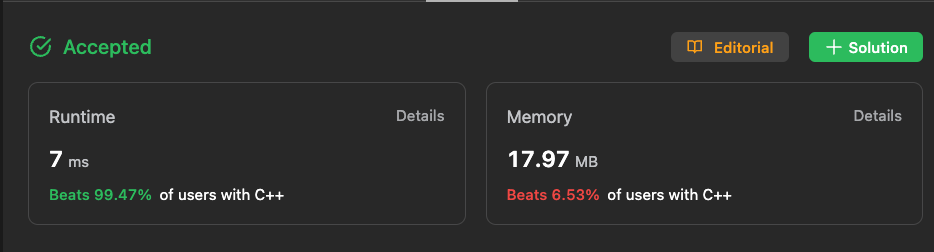
Here's the code that led us to this result.
Node* connect(Node* root) {
vector<vector<Node*>> levels;
connectHelper(root, 0, levels);
return root;
}
void connectHelper(Node *&root, int currentLevel, vector<vector<Node*>> &levels) {
if (root == NULL) return;
int nextLevel = currentLevel + 1;
if(levels.size() < nextLevel) levels.resize(nextLevel);
if( levels[currentLevel].size() > 0)
levels[currentLevel].back()->next = root;
levels[currentLevel].push_back(root);
connectHelper(root->left, nextLevel, levels);
connectHelper(root->right, nextLevel, levels);
}
Let's break down the code:
1. connect
is a function that takes a binary tree represented by the Node*
root
and returns the same root
after connecting the nodes at each level.
2. It initializes a vector of vectors called levels
to keep track of nodes at each level.
3. It calls the connectHelper
function to perform the actual connection.
4. connectHelper
is a recursive function used to traverse the binary tree and connect nodes at the same level.
5. It takes three parameters:
root
: A reference to a pointer to the current node in the traversal.currentLevel
: An integer representing the current level in the tree.levels
: A reference to the vector of vectors that stores nodes at each level.
6. If the root
is NULL
(indicating an empty subtree), the function returns early.
7. It calculates nextLevel
by incrementing currentLevel
by 1. This represents the level of the child nodes.
8. It ensures that the levels
vector has enough subvectors to accommodate nodes at the current level. If not, it resizes levels
accordingly.
9. If there are nodes in the current level (i.e., levels[currentLevel]
is not empty), it sets the next
pointer of the last node in that level to point to the current root
. This connects nodes at the same level.
10. It then adds the current root
to the current level's vector in levels
.
11. Finally, it recursively calls connectHelper
for the left and right child nodes, passing the nextLevel
and levels
vector by reference to continue the traversal and connection process.