LeetCode: 105. Construct Binary Tree from Preorder and Inorder Traversal
The Problem
Given two integer arrays preorder
and inorder
where preorder
is the preorder traversal of a binary tree and inorder
is the inorder traversal of the same tree, construct and return the binary tree.
Example
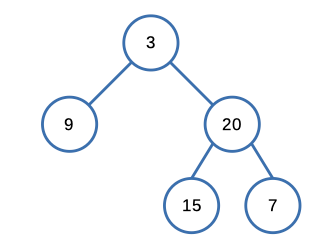
Input: preorder = [3,9,20,15,7], inorder = [9,3,15,20,7]
Output: [3,9,20,null,null,15,7]
Constraints:
1 <= preorder.length <= 3000
inorder.length == preorder.length
-3000 <= preorder[i], inorder[i] <= 3000
preorder
andinorder
consist of unique values.- Each value of
inorder
also appears inpreorder
. preorder
is guaranteed to be the preorder traversal of the tree.inorder
is guaranteed to be the inorder traversal of the tree.
Solution
We opted for a recursive approach to tackle this problem, and upon evaluating our solution on the LeetCode platform, we achieved the following outcome:
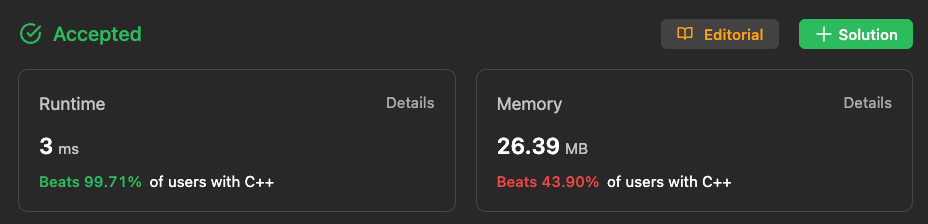
Here's the code that led us to this result.
TreeNode* buildTree(vector<int>& preorder, vector<int>& inorder) {
unordered_map<int, int> inorderIndexMap;
for (int i = 0; i < inorder.size(); ++i)
inorderIndexMap[inorder[i]] = i;
int preorderIndex = 0;
return buildTreeHelper(
preorder, inorder,
preorderIndex,
0, inorder.size() - 1,
inorderIndexMap
);
}
TreeNode* buildTreeHelper(
vector<int>& preorder, vector<int>& inorder,
int& preorderIndex,
int left, int right,
unordered_map<int, int>& inorderIndexMap
) {
if (left > right) return NULL;
int rootValue = preorder[preorderIndex];
TreeNode* root = new TreeNode(rootValue);
int inorderIndex = inorderIndexMap[rootValue];
++preorderIndex;
root->left = buildTreeHelper(
preorder, inorder,
preorderIndex,
left, inorderIndex - 1,
inorderIndexMap
);
root->right = buildTreeHelper(
preorder, inorder,
preorderIndex,
inorderIndex + 1, right,
inorderIndexMap
);
return root;
}
ðŸ§
Github with all the solution including test cases.
Explanation:
- The
buildTree
function is the entry point for constructing the binary tree. It takes thepreorder
andinorder
vectors as inputs. - An
unordered_map
namedinorderIndexMap
is created to store the indices of values in theinorder
array. This map will allow us to quickly find the position of a value in theinorder
traversal. - A
for
loop iterates through theinorder
array to populateinorderIndexMap
with values and their corresponding indices. - The
preorderIndex
variable is initialized to 0. It keeps track of the current position in thepreorder
array. - Finally, the
buildTreeHelper
function is called with the necessary parameters to construct the binary tree. - The
buildTreeHelper
function is a recursive function responsible for constructing a subtree of the binary tree. - It takes parameters including the
preorder
andinorder
vectors, thepreorderIndex
(used to track the position in thepreorder
array), and theleft
andright
indices (indicating the range of values in the current subtree). - The base case checks if the
left
index exceeds theright
index, in which case it returnsNULL
to indicate an empty subtree. - It retrieves the root value from the
preorder
array and creates a newTreeNode
with that value. - It finds the position of the root value in the
inorder
array usinginorderIndexMap
. - The
preorderIndex
is incremented to move to the next value in thepreorder
array. - The function then recursively constructs the left and right subtrees by adjusting the
left
andright
indices accordingly. - Finally, it returns the root of the constructed subtree.